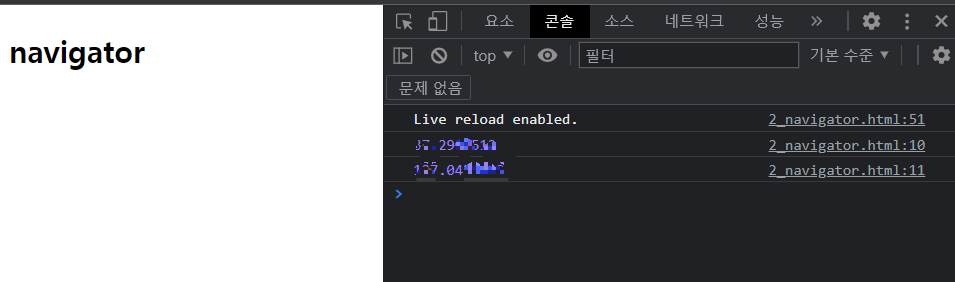
- history 객체
브라우저의 히스토리 정보를 문서와 문서 상태 목록으로 저장하는 객체이다.
사용자의 개인 정보를 보호하기 위해 이 객체의 대부분의 접근 방법을 제한한다.
함수 | 의미 |
back() | 뒤로 이동 |
forward() | 앞으로 이동 |
go(0) == location.reload() | 새로고침 |
- navigator 객체
브라우저 공급자 및 버전 정보등을 포함한 브라우저에 대한 정보를 저장하는 객체이다.
모바일에서는 GPS 정보를 갖고올 수 있다.
geolocation : GPS 정보를 수신하는 프로퍼티
navigator 객체 사용해보기!
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>navigator</title>
<script>
const success = function(loc) {
console.log(loc.coords.latitude);
console.log(loc.coords.longitude);
}
const fail = function(msg) {
console.log(mag.code);
}
navigator.geolocation.getCurrentPosition(success, fail);
</script>
</head>
<body>
<h2>navigator</h2>
</body>
</html>
현재 사용하는 와이파이 기지국의 위도와 경도를 출력하는 것을 확인 할 수 있다.
- 문서 객체 모델 (Document Object Model)
HTML 문서 또는 XML 문서 등을 접근하기 위한 일종의 인터페이스 역할 문서내의 모든 요소를 정의하고 각각의 요소에 접근하는 방법을 제공한다.
- document 객체
웹 페이지 자체를 의미하는 객체이다.
웹 페이지에 존재하는 HTML 요소에 접근하고자 할 때 반드시 document 객체로부터 시작해야한다.
함수 | 의미 |
getElementsByTagName() | 해당 태그 이름의 요소를 모두 선택한다. |
getElementById() | 해당 아이디의 요소를 선택한다. |
getElementsByClassName() | 해당 클래스에 속한 요소를 모두 선택한다. |
getElementsByName() | 해당 name 속성 값을 가지는 요소를 모두 선택한다. |
querySelectorAll(CSS선택자) | CSS 선택자로 선택되는 요소를 모두 선택한다. |
querySelector(CSS 선택) | CSS 선택자로 선택되는 요소를 선택한다. |
- 노드(node)
HTML DOM은 노드라고 불리는 계층적 단위에 정보를 저장하고 있다.
- 노드 종류
노드 | 의미 |
문서노드 | 문서 전체를 나타내는 노드이다. |
요소 노드 | HTML 요소는 요소노드, 속성 노드를 가질 수 있는 유일한 노드이다. |
속성 노드 | 속성은 모두 속성 노드이며, 요소 노드에 관한 정보를 가지고 있다. |
텍스트 노드 | 텍스트는 모두 텍스트 노드이다. |
주석 노드 | 주석은 모두 주석 노드이다. |
- 노드의 관계
parentNode | 부모노드 |
children | 자식 노드 리스트 |
childNodes | 자식 노드 리스트 |
firstChild | 첫 번째 자식 노드 |
firstElementChild | 첫 번째 자식 요소 노드 |
lastChild | 마지막 자식 노드 |
nextSibling | 다음 형제 노드 |
prevoiusSibling | 이전 형제 노드 |
- 노드 추가
appendChild() | 새로운 노드를 해당 노드의 자식 리스트 맨 마지막에 추가한다. |
insertBefore() | 새로운 노드를 특정 자식 노드 바로 앞에 추가한다, |
insertData() | 새로운 노드를 텍스트 데이터로 추가한다. |
- 노드 생성
createElement() | 새로운 요소 노드를 만든다. |
createAttribute | 새로운 속성 노드를 만든다. |
createTextNode() | 새로운 텍스트 노드를 만든다. |
- 노드 제거
removeChild() | 자식 노드 리스트에서 특정 자식 노드를 제거한다. 노드가 제거되면 해당 노드를 반환한다. |
removeAttribute() | 특정 속성 노드를 제거한다. |
- 노드 복제
cloneNode() | 기존의 존재하는 노드와 동일한 새로운 노드를 생성하여 반환한다. |
- 노드 교체
replacrChild() | 기존의 요소 노드를 새로운 요소 노드로 교체한다. |
replaceData() | 텍스트 노드의 텍스트 데이터를 교체한다. |
DOM 객체 및 node 사용해보기!
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>node</title>
<script>
function appendNode() {
const parent = document.getElementById('list');
const newItem = document.getElementById('item1');
parent.appendChild(newItem);
}
function insertNode() {
const parent = document.getElementById('list');
const backend = document.getElementById('backend');
const newItem = document.getElementById('item2');
parent.insertBefore(newItem, backend);
}
function appendText(){
const text = document.getElementById('text').firstChild;
text.insertData(7, ' 아주 피곤한 ')
console.log(text)
}
function createNode() {
const newItem = document.getElementById('item1');
const newNode = document.createElement('p');
newNode.innerHTML = '<b>새로운 요소가 나타났다!✨</b>';
document.body.insertBefore(newNode,newItem);
}
function createAttr() {
const newItem = document.getElementById('item2');
const newAttr = document.createAttribute('style');
newAttr.value = 'color : deeppink; background-color : aliceblue';
newItem.setAttributeNode(newAttr);
}
function createText() {
const textNode = document.getElementById('createText');
const newText = document.createTextNode('😍😎😁😀😊');
textNode.appendChild(newText);
}
function removeNode() {
const parent = document.getElementById('list');
const removeItem = document.getElementById('backend');
const result = parent.removeChild(removeItem);
console.log(result);
}
function removeAttr() {
const newItem = document.getElementById('item2');
newItem.removeAttribute('style');
}
function cloneElement() {
const parent = document.getElementById('list');
const originItem = document.getElementById('clone');
// originItem.cloneElement(true); // true : 자식 노드 까지 복사
parent.appendChild(originItem.cloneNode(true));
}
function changeNode() {
const parent = document.getElementById('list');
const item1 = document.getElementById('backend');
const item2 = document.getElementById('item1');
parent.replaceChild(item2, item1);
}
function changeText() {
const text = document.getElementById('fruit').firstChild;
text.replaceData(9, 2, '메론');
}
</script>
</head>
<body>
<h2 id="clone">노드</h2>
<p id="item1">JavaScript</p>
<p id="item2">React</p>
<hr>
<div id="list">
<p id="backend">node.js</p>
<p>HTML</p>
<p>CSS</p>
</div>
<hr>
<pd id="text"> 현재 시간은 오전 11시 10분입니다.</pd>
<hr>
<p id = "createText"></p>
<hr>
<p id="fruit">좋아하는 과일은 딸기입니다.</p>
<hr>
<button onclick = "appendNode()">노드 추가 1</button>
<button onclick="insertNode()">노드 추가 2</button>
<button onclick="appendText()">텍스트 추가</button>
<button onclick="createNode()">요소 노드 추가</button>
<button onclick="createAttr()">속성 노드 추가</button>
<button onclick="createText()">텍스트 노드 추가</button>
<button onclick="removeNode()">요소 노드 삭제</button>
<button onclick="removeAttr()">속성 노드 삭제</button>
<button onclick="cloneElement()">노드 복제</button>
<button onclick="changeNode()">요소 노드 교체</button>
<button onclick="changeText()">텍스트 노드 교체</button>
</body>
</html>
- 정규 표현식
정규 표현식 또는 정규식은 문자열에서 특정 문자 조합을 찾기 위한 패턴이다.
/ ~ / | 시작과 끝 |
[ ] | 시작 |
[x-z] | 안의 문자를 찾음 |
x+ | x가 1번이상 반복 |
x$ | 문자열이 x로 끝남 |
^x | 문자열이 x로 시작 |
\d | 숫자 |
x{n} | x를 n번 반복한 문자를 찾음 |
x{n,m} | x를 n번 이상 m번 이하 반복한 문자를 찾음 |
✔ test() 함수
정규 표현식에 대입한 문자열이 적합하면 true, 아니면 false
'국비 > JavaScript' 카테고리의 다른 글
DAY 03 - 1 : 화살표 함수, 객체, 상속 (0) | 2022.11.14 |
---|---|
DAY 02 -2 : 배열, 향상된 for문, 호이스팅, 함수 (0) | 2022.11.14 |
DAY 02 - 1 : 대화상자, 연산자, 제어문(조건문, 반복문) (0) | 2022.11.07 |
DAY 01 : JavaScript, 변수, 데이터타입, 형변환, .. (0) | 2022.11.06 |